I built a bot that can play wordle with a better than 98% success rate.
Wordle is a surprisingly competitive game about guessing the correct word within 6 guesses. When I noticed the game was getting very popular I decided to try for myself. I sucked. Sometimes I would get so stuck that sometimes I would use online wordle solvers. I thought that building one of my own would be a good exercise, and sure enough, after experimenting for 3 hours, I had a working prototype. After hours of testing, I got bored of typing in (specially formatted) board states into a command line and wanted to build a new, fully automated version that would play the game for you.
How does it work?
Web interface
As mentioned in the introduction, wordlebot uses selenium to simulate inputs to the wordle+ web page, allowing it to read the current game state (the colouring of each of the letter tiles) and input it’s generated guesses into the site, as well as detecting a win or lose.
Dictionaries
- It uses any dictionary you give it, so you can swap out your dictionaries for better ones
- If wordlebot finds more than 1 optimal word, it chooses the highest one in the dictionary, so that dictionaries can be sorted from most common to least common
- Wordlebot builds on the dictionary, adding new words that weren’t in the dictionary yet, and rearranging the dictionary to better represent the most to least common words
- If a word is not accepted, wordlebot “burns” it, deleting it
Wordle Algorithm
- There are many wordle algorithms out there. The algorithm wordlebot uses is by far not the best, but I use it because it was relatively easy to implement.
- Wordlebot’s code contains a list of letters; each one assigned a point correlating to their probability in percentage (e.g ~11% of letters in English are the letter e, so e gets given 11 points)
- Each word is given points by adding up all of it’s individual letter’s points (note that duplicate letters don’t count)
- The algorithm works by going through all valid words in its dictionary’s (from most to least common) and finding the 4 highest scoring words
- The final guess word is chosen by being the highest in the list (most common)
Logging
After each game, wordlebot logs the result of the game, the logs are formatted like this:
- A win: {correct word},{number of guesses},{date}/{month}/{year},{hour}:{month}:{second}
- A loss {correct word},FAIL,{date}/{month}/{year},{hour}:{month}:{second}
- Invalid word {invalid word},FAIL(wrdNAcpt),{date}/{month}/{year},{hour}:{month}:{second}
- No valid word {correct word},FAIL(nVladDict),{date}/{month}/{year},{hour}:{month}:{second}
- Crash @{date}/{month}/{year},{hour}:{month}:{second} \n {error}
Challenges in building wordlebot
Web interface
Seeing as most sites (including wordle+) are not designed for bot use – many even intentionally obfuscating their web pages to prevent scraping – building the web interface for wordlebot was not fun
Locating Elements
Perhaps the most complicated parts of building wordlebot, XPaths (the most common way of locating specific elements on a webpage) easily break when only one element changes in the path.
Complicating this further, when multiple variations of an element exist (for example the correct word for a wordle, that sometimes has a different path if it’s definition could not be found) this meant that I would have to leave wordlebot running for a while until it crashed, where I would have to find the element that caused the crash, fix the XPath responsible for locating it and leave it to run again until I found the next error, so on and so forth.
Auto Log and reboot
If an error occurs, wordlebot is programmed to log it, and then reboot, this is in case of edge cases (either relating to the algorithm or web interface) that I hadn’t discovered and patched.
Results!
After building wordlebot, I decided to test it by running it on 20,000 wordle games starting on 1 word, “first”, and building on it. Here are the results:
- After 20,392 games, wordlebot’s dictionary was 2,352 words long
- The average win rate was approximately 98.73%
Video
Here is a video of wordlebot running on my laptop.
Graphs
I converted the outputted stats.txt to a CSV, which I gave to ChatGPT to generate these graphs:
Game Outcomes:
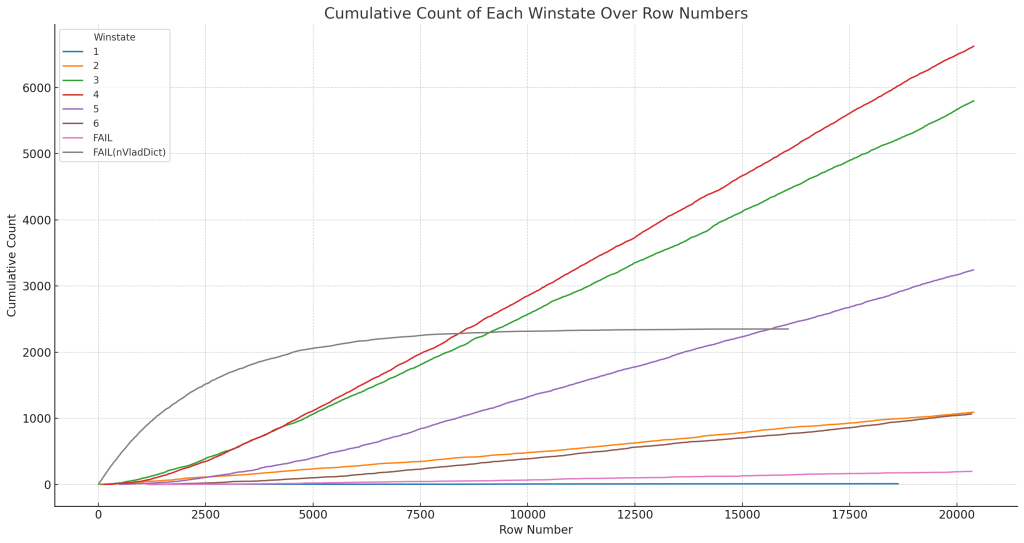
Cumulative Win Rate:
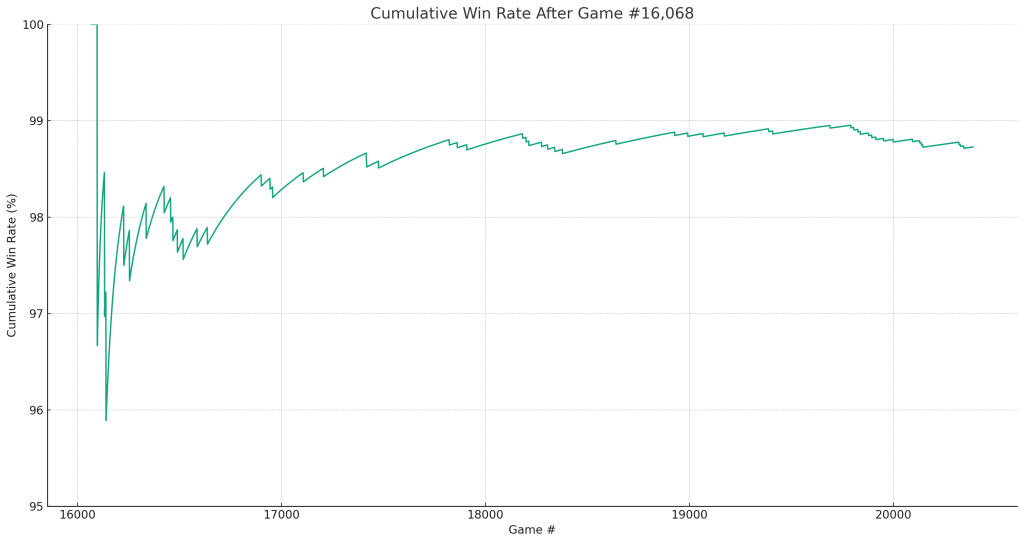
Note
To prevent people spamming wordle+, the open-source version of wordlebot has had all Selenium automations removed. Wordlebot still uses the same algorithm, but instead of a Selenium interface, it uses a CLI for the user to input the current game state.